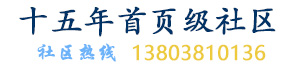
精通
英语
和
开源
,
擅长
开发
与
培训
,
胸怀四海
第一信赖
锐英源精品开源心得,转载请注明:“锐英源www.wisestudy.cn,孙老师作品,电话13803810136。”需要全文内容也请联系孙老师。
Introduction
My C++ programming background comes from DOS Borland C++. In their latest DOS version 3.1, they included a template library for collections. It was a great piece of work. When I started to use Visual C++ v2.2, I even tried to use Borland's collection template library within Visual C++ but with no success. The only other solution was to switch to Microsoft's collections that are part of the MFC. However, this was always a problem for the following reasons:
Recently, I started using STL and it is great. The beginning was a bit difficult but once I grasped the idea, it was easy. This article contains some first hand experiences with STL and is intended for
programmers that want to use STL fast and without going into greater details.
我的C + +编程背景从DOS下Borland公司的C + +。在其最新的DOS版本3.1,它们包括一个集合的模板库。这是一个很大的一块工作。当我开始使用Visual C + + V2.2,我甚至尝试使用在Visual C + +,但没有成功地在VC++下面用Borland的模板库。唯一的解决办法是切换到微软的MFC的集合。然而,这总是一个问题,原因如下:
最近,我开始使用STL,它是伟大的。刚开始是有点困难,但一旦我掌握了的想法,很容易。本文包含一些第一手经验,让想用STL程序员能够快速使用STL,并不会被细节给困扰。
Rule 1:
You can create STL containers that store either objects or pointer to objects.
您可以创建STL容器存储对象或对象的指针。
class TMyClass; typedef list<TMyClass> TMyClassList; // Stores objects into the list container typedef list<TMyClass*> TMyClassPtrList; // Stores pointers to object into the list container
Usually, list container that stores the object is used. However, if the object is using a machine resource (handle to file, named pipe, socket or similar), then it is more appropriate to use lists that stores pointers to objects.
If the container stores objects, then it is automatically cleaned up during container destruction. However, if it stores pointers to objects,
programmer is responsible to delete all pointers.
通常情况下,list容器用于存储对象。但是,如果对象是使用一台机器的资源(处理文件,命名管道,SOCKET或类似),然后这是比较适合让列表存储对象的指针。
如果容器存储对象,那么它会自动在容器销毁时清理对象。但是,如果它存储的对象的指针,程序员负责删除所有的指针。
Rule 2:
Each class (whose instance will go into the container) must implement at least the copy constructor (it is good to implement also the assignment operator.
每个类(其实例要在容器内使用)必须至少实现拷贝构造函数(实现赋值运算符,也是好习惯)。
class TMyClass { private: ... public: TMyClass(..); // Copy constructor TMyClass(const TMyClass& obj) { *this = obj; } // Assignment operator TMyClass& operator=(const TMyClass& obj); ... };
This is necessary since the STL will create a local copy of the object when you insert an object instance into the container. If you do not write a correct code for the copy constructor, object within a list will have some data members uninitialized.
当你向容器中插入一个对象的实例,STL将创建一个对象的本地副本,所以说上面处理是必要的。如果你不写一个拷贝构造函数,在一个列表中的对象,将有一些数据成员没有初始化。
Rule 3:
Inserting an object into the container is done in the following way:
以下列方式把一个对象插入到容器中,:
TMyClass object; TMyClassList myList; TMyClassList::iterator it; it = myList.insert(myList.end(), object); TMyClass *pObject = &(*it);
Previous example shows how to insert an object into the container and obtain a pointer to the object within container. This is necessary since the container will create a new copy of the "object" instance and the original "object" instance is not used any more. In case you are storing pointers to a list, this is not necessary since original pointer is stored into the container.
前面的例子说明如何插入一个对象到容器中,并获得一个指向容器内的对象的指针。这是必要的,因为容器将创建一个新的“对象”实例的副本,且原来的“对象”的实例是不再使用了。如果你是存储到一个列表中的指针,这是没有必要,因为原来的指针也放入容器中存储了。
Rule 4:
Iterating through container is done in the following way:
通过容器的迭代以下列方式进行:
TMyClassList::iterator it; TMyClass *pObject; for (it = myList.begin(); it != myList.end(); it ++) { pObject = &(*it); // Use pObject }
However, if you are storing pointers into the container, then the previous code fragment has to be modified to the following:
不过,如果你把指针存储放入到容器内,然后在前面的代码片段进行如下修改: